728x90
반응형
import tensorflow as tf
img = tf.keras.utils.get_file('zebra.jpg','https://i.imgur.com/XjeiRMV.jpg')
import cv2
import matplotlib.pyplot as plt
im = cv2.imread(img)
im = cv2.cvtColor(im, cv2.COLOR_BGR2RGB)
im_ = im.copy()
rec1 = cv2.rectangle(im_, (120,25),(200,165), color=(255,0,0), thickness=2)
rec2 = cv2.rectangle(im_, (300,50),(480,320), color=(255,0,0), thickness=2)
plt.imshow(im_)
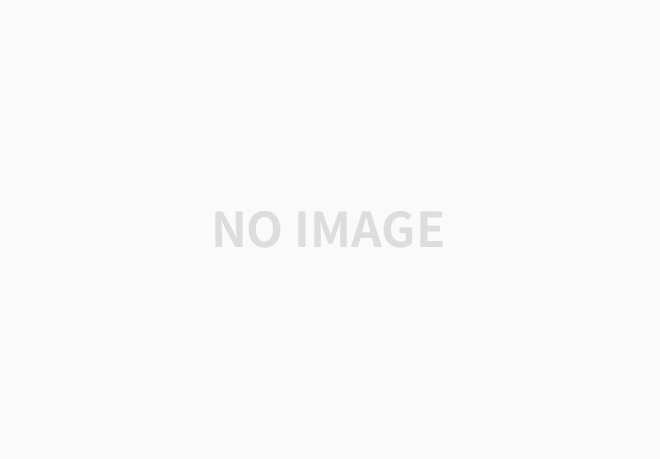
im_.shape
# (333, 500, 3)
h = w = 800
im_r = cv2.resize(im, (h,w))
im_r_ = im_r.copy()
import numpy as np
x = np.array([120, 25, 200, 165])
y = np.array([300, 50, 480,320])
x[0] = int(x[0]*(w/im.shape[1]))
x[1] = int(x[1]*(h/im.shape[0]))
x[2] = int(x[2]*(w/im.shape[1]))
x[3] = int(x[3]*(h/im.shape[0]))
y[0] = int(y[0]*(w/im.shape[1]))
y[1] = int(y[1]*(h/im.shape[0]))
y[2] = int(y[2]*(w/im.shape[1]))
y[3] = int(y[3]*(h/im.shape[0]))
rec1 = cv2.rectangle(im_r_, (x[0],x[1]),(x[2],x[3]), color=(255,0,0), thickness=2)
rec2 = cv2.rectangle(im_r_, (y[0],y[1]),(y[2],y[3]), color=(255,0,0), thickness=2)
from skimage.util import view_as_blocks, view_as_windows
plt.figure(figsize=(8,8))
plt.imshow(im_r_)
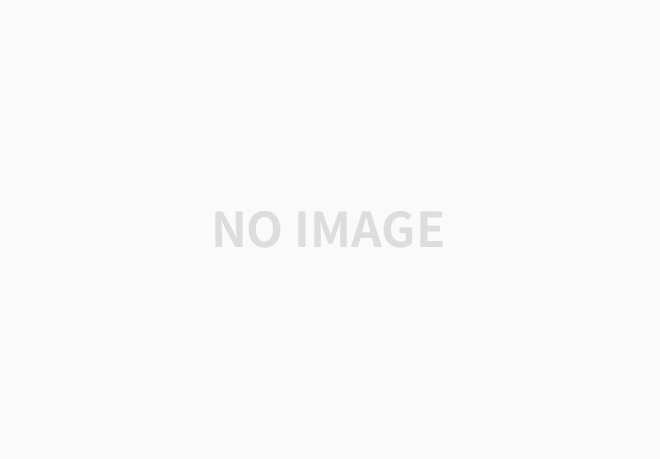
vgg = tf.keras.applications.VGG16(include_top=False)
for j,i in enumerate(vgg.layers):
output = tf.keras.models.Model(vgg.input, i.output)
print(output(im_r_[tf.newaxis]).shape,j)
(1, 800, 800, 3) 0
(1, 800, 800, 64) 1
(1, 800, 800, 64) 2
(1, 400, 400, 64) 3
(1, 400, 400, 128) 4
(1, 400, 400, 128) 5
(1, 200, 200, 128) 6
(1, 200, 200, 256) 7
(1, 200, 200, 256) 8
(1, 200, 200, 256) 9
(1, 100, 100, 256) 10
(1, 100, 100, 512) 11
(1, 100, 100, 512) 12
(1, 100, 100, 512) 13
(1, 50, 50, 512) 14
(1, 50, 50, 512) 15
(1, 50, 50, 512) 16
(1, 50, 50, 512) 17
(1, 25, 25, 512) 18
backbone = tf.keras.models.Model(vgg.input, vgg.layers[17].output)
backbone(im_r_[tf.newaxis]).shape
# TensorShape([1, 50, 50, 512])
plt.imshow(backbone(im_r_[tf.newaxis])[0,...,4])
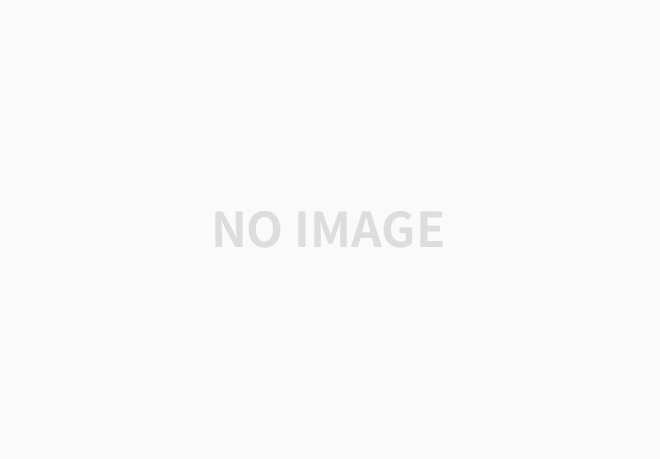
vgg.summary()
Model: "vgg16"
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
input_3 (InputLayer) [(None, None, None, 3)] 0
_________________________________________________________________
block1_conv1 (Conv2D) (None, None, None, 64) 1792
_________________________________________________________________
block1_conv2 (Conv2D) (None, None, None, 64) 36928
_________________________________________________________________
block1_pool (MaxPooling2D) (None, None, None, 64) 0
_________________________________________________________________
block2_conv1 (Conv2D) (None, None, None, 128) 73856
_________________________________________________________________
block2_conv2 (Conv2D) (None, None, None, 128) 147584
_________________________________________________________________
block2_pool (MaxPooling2D) (None, None, None, 128) 0
_________________________________________________________________
block3_conv1 (Conv2D) (None, None, None, 256) 295168
_________________________________________________________________
block3_conv2 (Conv2D) (None, None, None, 256) 590080
_________________________________________________________________
block3_conv3 (Conv2D) (None, None, None, 256) 590080
_________________________________________________________________
block3_pool (MaxPooling2D) (None, None, None, 256) 0
_________________________________________________________________
block4_conv1 (Conv2D) (None, None, None, 512) 1180160
_________________________________________________________________
block4_conv2 (Conv2D) (None, None, None, 512) 2359808
_________________________________________________________________
block4_conv3 (Conv2D) (None, None, None, 512) 2359808
_________________________________________________________________
block4_pool (MaxPooling2D) (None, None, None, 512) 0
_________________________________________________________________
block5_conv1 (Conv2D) (None, None, None, 512) 2359808
_________________________________________________________________
block5_conv2 (Conv2D) (None, None, None, 512) 2359808
_________________________________________________________________
block5_conv3 (Conv2D) (None, None, None, 512) 2359808
_________________________________________________________________
block5_pool (MaxPooling2D) (None, None, None, 512) 0
=================================================================
Total params: 14,714,688
Trainable params: 14,714,688
Non-trainable params: 0
_________________________________________________________________
vgg(im_r_[tf.newaxis])
<tf.Tensor: shape=(1, 25, 25, 512), dtype=float32, numpy=
array([[[[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0.142358 , ..., 0. ,
0. , 0. ],
...,
[ 0. , 0. , 1.3040222 , ..., 0. ,
2.3414693 , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
3.648667 , 0. ],
[ 0. , 0. , 2.5827253 , ..., 0. ,
1.2787921 , 0. ]],
[[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
...,
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ]],
[[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
...,
[ 0. , 0. , 25.991032 , ..., 0. ,
4.2155175 , 0. ],
[ 0. , 0. , 9.656704 , ..., 0. ,
6.1238546 , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ]],
...,
[[ 0. , 0. , 0. , ..., 0. ,
2.6076157 , 0.24721637],
[ 0. , 0. , 0. , ..., 0. ,
0. , 1.4595927 ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0.13756028],
...,
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ]],
[[15.054876 , 0. , 0. , ..., 0. ,
2.182668 , 0. ],
[11.117934 , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
...,
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ]],
[[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0.29810184, 0. ],
[ 0. , 0. , 0. , ..., 0. ,
1.1234534 , 0. ],
...,
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ],
[ 0. , 0. , 0. , ..., 0. ,
0. , 0. ]]]], dtype=float32)>
im_r = cv2.resize(im, (h,w))
im_r_ = im_r.copy()
x = np.arange(8,800,16)
y = np.arange(8,800,16)
cl = np.array(np.meshgrid(x,y)).T.reshape(-1,2)
for i in range(2500):
cv2.circle(im_r_, (cl[i,0], cl[i,1]),1, (255,0,0), thickness=2)
plt.figure(figsize=(10,10))
plt.imshow(im_r_)
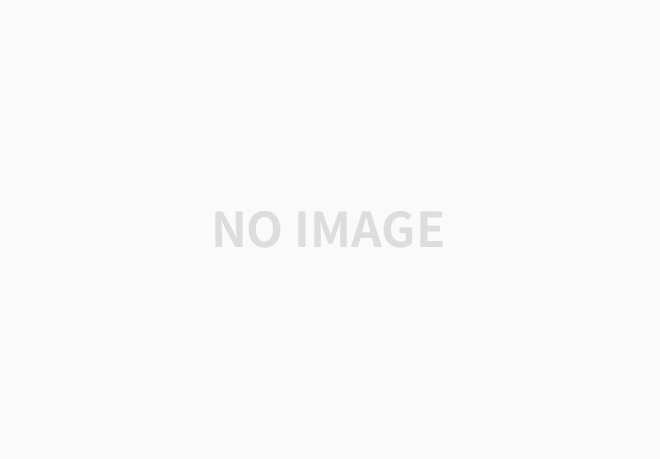
50*50*9
# 22500
ratio = [0.5, 1, 2]
scale = [8,16,32]
al = np.zeros((22500,4))
count = 0
for i in cl:
cx, cy = i[0],i[1]
for r in ratio:
for s in scale:
h = pow(pow(s,2)/r,0.5)
w = h*r
h *= 16
w *= 16
xmin = cx-0.5*w
ymin = cy-0.5*h
xmax = cx+0.5*w
ymax = cy+0.5*h
al[count] = [xmin, ymin,xmax,ymax]
count += 1
al.shape
# (22500, 4)
point = 570
im_r_
array([[[ 87, 51, 37],
[ 91, 52, 40],
[ 97, 54, 45],
...,
[ 75, 48, 37],
[ 67, 45, 35],
[ 61, 43, 33]],
[[ 87, 51, 37],
[ 91, 53, 40],
[ 97, 54, 45],
...,
[ 74, 47, 36],
[ 66, 44, 34],
[ 60, 42, 32]],
[[ 86, 52, 38],
[ 90, 53, 41],
[ 95, 54, 44],
...,
[ 70, 43, 32],
[ 62, 40, 30],
[ 56, 38, 29]],
...,
[[153, 93, 65],
[106, 63, 43],
[ 49, 28, 16],
...,
[ 85, 50, 28],
[129, 84, 55],
[166, 113, 78]],
[[106, 59, 47],
[ 95, 50, 37],
[ 82, 41, 25],
...,
[108, 75, 51],
[115, 75, 51],
[123, 78, 53]],
[[ 92, 49, 42],
[ 91, 46, 35],
[ 91, 45, 28],
...,
[114, 83, 58],
[110, 73, 50],
[110, 68, 46]]], dtype=uint8)
# img_ = np.copy(im_r)
# for i in range(point,point+9):
# x_min = int(al[i][0])
# y_min = int(al[i][1])
# x_max = int(al[i][2])
# y_max = int(al[i][3])
# cv2.rectangle(img_, (x_min,y_min),(x_max,y_max), (0,255,0), thickness=4)
# for i in range(2500):
# cv2.circle(img_, (cl[i,0], cl[i,1]),1, (0,0,255), thickness=2)
x = np.array([120, 25, 200, 165])
y = np.array([300, 50, 480,320])
x[0] = int(x[0]*1.6)
x[1] = int(x[1]*2.4)
x[2] = int(x[2]*1.6)
x[3] = int(x[3]*2.4)
y[0] = int(y[0]*1.6)
y[1] = int(y[1]*2.4)
y[2] = int(y[2]*1.6)
y[3] = int(y[3]*2.4)
rec1 = cv2.rectangle(im_r_, (x[0],x[1]),(x[2],x[3]), color=(255,0,0), thickness=5)
rec2 = cv2.rectangle(im_r_, (y[0],y[1]),(y[2],y[3]), color=(255,0,0), thickness=5)
plt.imshow(im_r_)
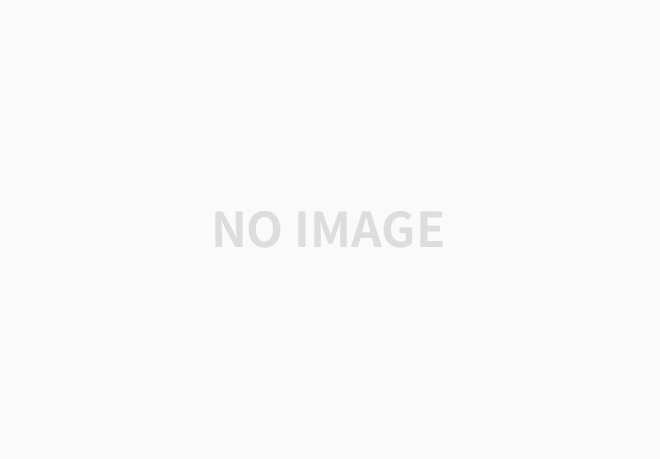
22500 > 0< 800> 제외
np.where((al[:,0] >=0) & (al[:,1] >=0) & (al[:,2] <= 800 ) & (al[:,3] <= 800 ))
# (array([ 1404, 1413, 1422, ..., 21069, 21078, 21087], dtype=int64),)
is_al = al[np.where((al[:,0] >=0) & (al[:,1] >=0) & (al[:,2] <= 800 ) & (al[:,3] <= 800 ))]
len(is_al) # anchor
# 8940
def iou(box1,box2):
x1 = max(box1[0], box2[0])
y1 = max(box1[1], box2[1])
x2 = min(box1[2], box2[2])
y2 = min(box1[3], box2[3])
if (x1 < x2 and y1 < y2):
w_o = x2 - x1
h_o = y2 - y1
area = w_o*h_o
else:
return 0
area_b1 = (box1[2]-box1[0])*(box1[3]-box1[1])
area_b2 = (box2[2]-box2[0])*(box2[3]-box2[1])
union = area_b1 + area_b2 - area
return area/union
object 1 = x
x = np.array([300, 50, 480,320])
x[0] = int(x[0]*1.6)
x[1] = int(x[1]*2.4)
x[2] = int(x[2]*1.6)
x[3] = int(x[3]*2.4)
object 2 = y
y = np.array([120, 25, 200, 165])
y[0] = int(y[0]*1.6)
y[1] = int(y[1]*2.4)
y[2] = int(y[2]*1.6)
y[3] = int(y[3]*2.4)
objects = [x,y]
result = np.zeros((8940,len(objects)))
for t,g in enumerate(objects):
for i,j in enumerate(is_al):
result[i][t] = iou(j,g)
result
array([[0. , 0. ],
[0. , 0. ],
[0. , 0. ],
...,
[0.06581804, 0. ],
[0.06484636, 0. ],
[0.05869298, 0. ]])
anchor_id = np.where((al[:,0] >=0) & (al[:,1] >=0) & (al[:,2] <= 800 ) & (al[:,3] <= 800 ))
anchor_id[0]
# array([ 1404, 1413, 1422, ..., 21069, 21078, 21087], dtype=int64)
pandas : 2차원
data = pd.DataFrame(data=[anchor_id[0], result[:,0], result[:,1]]).T
data.rename(columns={0:'anchor_id', 1:'o1_iou',2:'o2_iou'}, inplace=True)
data.anchor_id = data.anchor_id.astype('int')
data
anchor_id o1_iou o2_iou
0 1404 0.000000 0.0
1 1413 0.000000 0.0
2 1422 0.000000 0.0
3 1431 0.000000 0.0
4 1440 0.000000 0.0
... ... ... ...
8935 21051 0.065818 0.0
8936 21060 0.065818 0.0
8937 21069 0.065818 0.0
8938 21078 0.064846 0.0
8939 21087 0.058693 0.0
data['o1_iou_objectness'] = data.apply(lambda x: 1 if x['o1_iou'] > 0.7 else -1, axis=1)
data[data['o1_iou_objectness'] == 1]
anchor_id o1_iou o2_iou o1_iou_objectness
7540 16877 0.711914 0.0 1
7547 16886 0.711914 0.0 1
7768 17327 0.711914 0.0 1
7775 17336 0.711914 0.0 1
data.o2_iou.argmax()
# 1785
data.loc[data.o2_iou.argmax()]
anchor_id 6418.00000
o1_iou 0.00000
o2_iou 0.65625
o1_iou_objectness -1.00000
Name: 1785, dtype: float64
top
array([[418.98066402, 45.96132803, 781.01933598, 770.03867197],
[418.98066402, 61.96132803, 781.01933598, 786.03867197],
[434.98066402, 45.96132803, 797.01933598, 770.03867197],
[434.98066402, 61.96132803, 797.01933598, 786.03867197]])
img_ = np.copy(im_r)
for i,j in enumerate(top):
x_min = int(top[i][0])
y_min = int(top[i][1])
x_max = int(top[i][2])
y_max = int(top[i][3])
cv2.rectangle(img_, (x_min,y_min),(x_max,y_max), (0,255,0), thickness=1)
# x = np.array([120, 25, 200, 165])
# y = np.array([300, 50, 480,320])
# x[0] = int(x[0]*1.6)
# x[1] = int(x[1]*2.4)
# x[2] = int(x[2]*1.6)
# x[3] = int(x[3]*2.4)
# y[0] = int(y[0]*1.6)
# y[1] = int(y[1]*2.4)
# y[2] = int(y[2]*1.6)
# y[3] = int(y[3]*2.4)
# # rec1 = cv2.rectangle(im_r_, (x[0],x[1]),(x[2],x[3]), color=(255,0,0), thickness=5)
# # rec2 = cv2.rectangle(im_r_, (y[0],y[1]),(y[2],y[3]), color=(255,0,0), thickness=5)
plt.figure(figsize=(10,10))
plt.imshow(img_)
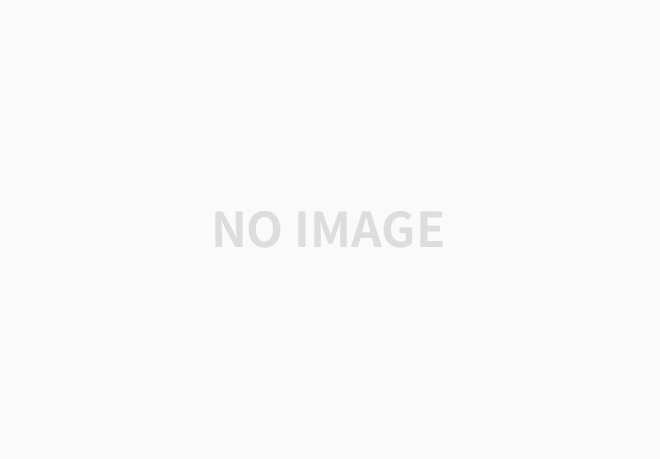
반응형
'Computer_Science > Visual Intelligence' 카테고리의 다른 글
31일차 - 논문 수업 (Detection) (0) | 2021.11.01 |
---|---|
30일차 논문 수업 (Detection) (0) | 2021.11.01 |
29일차 - 논문 수업 (Detection) (0) | 2021.10.24 |
28일차 - 논문 수업 (Detection) (0) | 2021.10.24 |
27일차 - 논문 수업 (Detection) (0) | 2021.10.24 |